Lua Script Examples
These snippets would be used as part of the code in an event initiated by a track contact.
Lua script to stop a tram then turn it round
(or you can use a depot)
if not deferredCall then
if vehicle:hasEngine() and direction == 1 then
vehicle.targetSpeed = 0
defer(5, "Turn around")
end
elseif deferredCall == "Turn around" then
-- rotate the vehicle by 180 degrees
vehicle.transformation:rotateZ(math.pi)
vehicle.targetSpeed = 20
end
Lua script to stop a passenger train but not a goods train
local vehicles = layout:getVehicleGroup(vehicle)
local train_has_coaches = false
if #vehicles then
for index = 1, #vehicles do
if vehicles[index].name == "Corridor Coach" then
train_has_coaches = true
end
if vehicles[index].name == "Compartment Coach" then
train_has_coaches = true
end
end
if train_has_coaches then
vehicle.currentSpeed = 0
end
end
Lua script to animate an object
Format:
layout:getEntityByName("object-name").animations["animation-name"]:play(a, b)
Where:
object-name is set in the object's properties panel
animation-name is set in the object's .anim text file
a =
-1 means current state of the animation
0 means start of the animation
1 means end of the animation
b =
1 means play the animation forward
-1 means play the animation backwards
Example:
layout:getEntityByName("Signal T1").animations["home-signal"]:play(-1,-1)
Direction
A track contact event will fire when a vehicle goes over a contact. Sometimes you’ll want to know which way the vehicle is travelling. The direction of travel is available in the variable: direction.
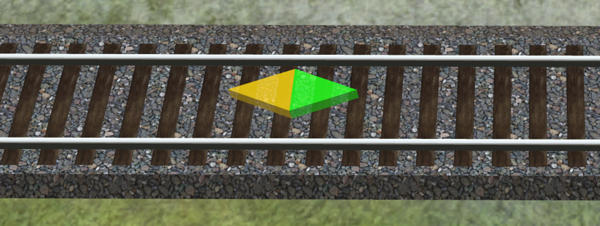
The variable direction can be either 1 or -1, so we could write an event handler script like this:
if direction == 1 then
-- vehicle is travelling in the direction of the green arrow
end
if direction == -1 then
-- vehicle is travelling in the direction of the yellow arrow
end
The orientation of the engine is not relevant. So an engine going backwards from left to right on the track in the picture would have a direction value of 1.